Deserializing JSON in Swift 5 and Contentful
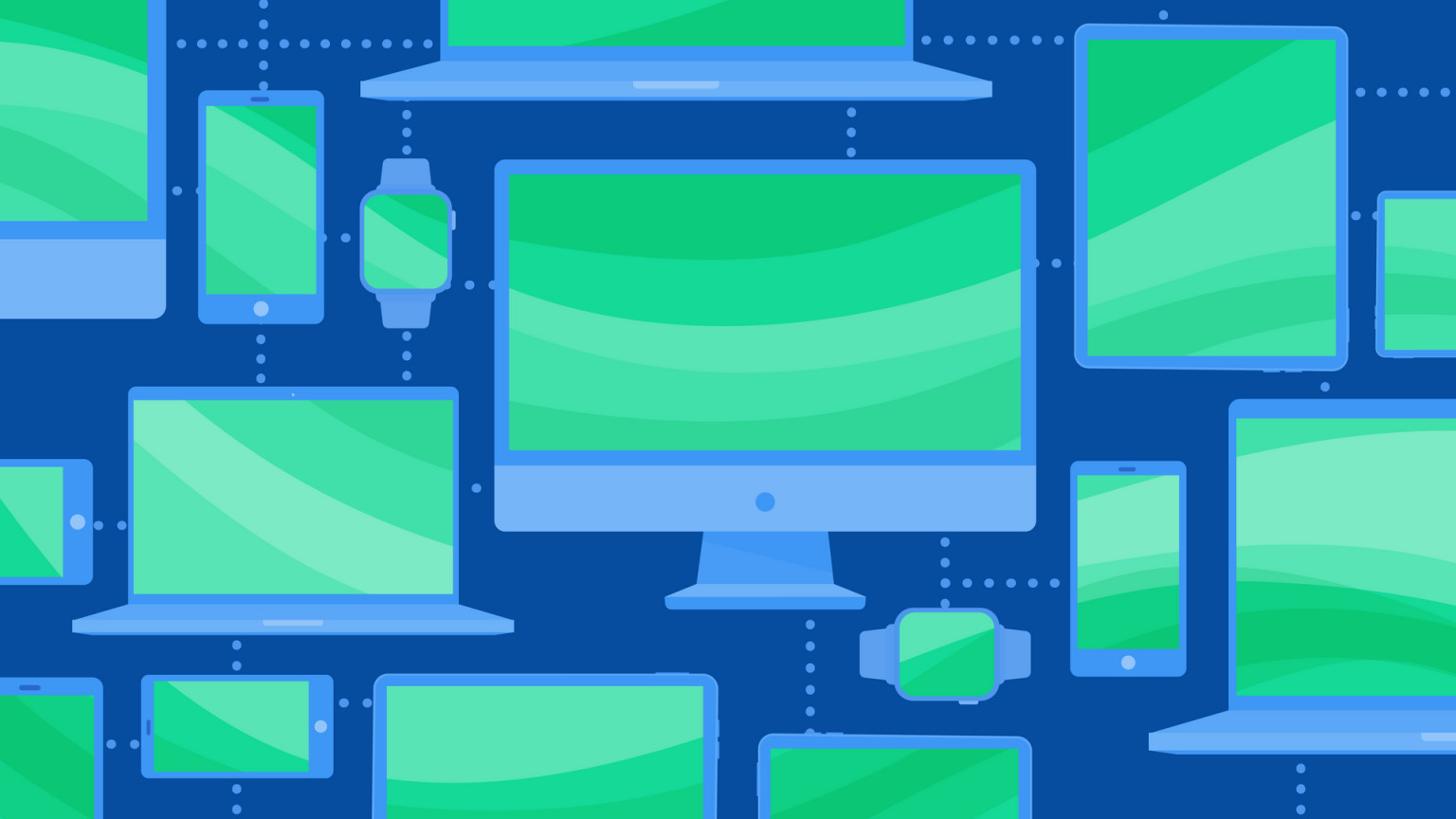
The internet requires businesses to create content that’s constantly available to customers to stay competitive — or even afloat. But with this comes a significant challenge: building applications and content that are interoperable across frameworks and tech stacks.
Fortunately, the JSON format paired with Contentful enables developers to form the building blocks of such platforms. It also works seamlessly with Swift, the officially supported programming language for iOS development, though it’s also available for Linux, Windows, and macOS. Swift enables you to deserialize JSON data with Apple’s Foundation APIs.
The latest version, Swift 5, has significantly upgraded the platform and development experience for Apple app developers. It provides many benefits over the legacy Objective-C frameworks and Swift 4, including:
Dynamic callable types.
Raw strings, enabling developers to write human-readable text and punctuation without escaping the literal.
Strict null-correctness, using the nil value in your programs to avoid memory leaks.
Introducing binary compatibility, ensuring the future releases of the Swift platform remain compatible with Swift 5.
The initial versions of Swift focused on interoperability with Objective-C and compatibility. That helped developers move their applications from Objective-C to Swift quickly.
Swift 5 has an ability to deserialize JSON. The deserialization process involves converting the JSON data from the string format to the runtime representation of objects. While serialization enables you to store the data for a program, deserialization enables you to restore the state using a backed-up instance.
When you’re using external APIs, like those available with Contentful, to deliver content to apps, websites, and other media, you deliver content in JSON form. This article walks you through using Swift 5 to process JSON data and deserialize it to use in programs. Then, you’ll learn how to make requests to Contentful APIs and deserialize the data to render the results.
Handling JSON in Swift
JSON is a standard of data interchange for all modern programming languages and can serve as a text representation of the state of your data. Converting your program data to text is called serialization. The opposite process, converting text to program data, is called deserialization. In the Swift SDK, these processes are known as encoding and decoding.
The benefits of using the JSON interchange format are:
JSON is a human-readable format.
Every framework and platform has built-in support for the JSON data type.
You can represent almost everything that your program contains in a JSON document.
JSON is a well-structured standard.
JSON deserialization basics
A JSON document treats everything as an object or a collection. An object can be a value or a key/value pair, and a collection can be a list of objects.
If you’re building client-facing applications, you’ll find yourself deserializing/decoding JSON documents. This is because Web APIs often provide consumable content in JSON format. You take this human-readable JSON content and deserialize it into machine-readable objects.
The earlier versions of Swift used the JSONSerialization type, which is used for interoperability between Objective-C and Swift code. The current Swift version uses the JSONDecoder, which only requires that your data type conforms to the Codable protocol.
JSONDecoder is the type that you can use to deserialize a JSON document to Swift objects of a specific type. Let’s explore some non-array examples of JSON decoding in Swift.
JSON data
Although a JSON document is used to represent objects and collections, a JSON document can also contain a scalar value, such as a string or an integer. These scalar values can be used as scalar inputs to parameters or as a value on the screen.
However, this isn’t scalable because a scalar value doesn’t represent the complete state of an operation or the server. This is because a JSON document either contains an object with the complete state or a collection of objects, each representing a record of information.
JSON objects
A JSON object is represented as a JavaScript object and can contain any number of properties, also known as key-value pairs. The following is an example of a JSON document with three properties: id
, name
, and age
.
The fields id and age have a value of type integer, while the field name has a value of type string. To deserialize this data using Swift 5, you can first create a type representing this data in memory as an object.
Note that the names of the fields in the JSON document match the names of the properties in your person type. If the names don’t match the document and the object, you need to provide CodingKeys in the Codable type. Using CodingKeys is beyond the scope of this article, but take note of this for your own projects.
After you’ve created the type, you can use the JSONDecoder to deserialize the data and print the information on the screen.
You can use any Swift IDE to run the code. The output of this program in Xcode Playground is shown in the image below:
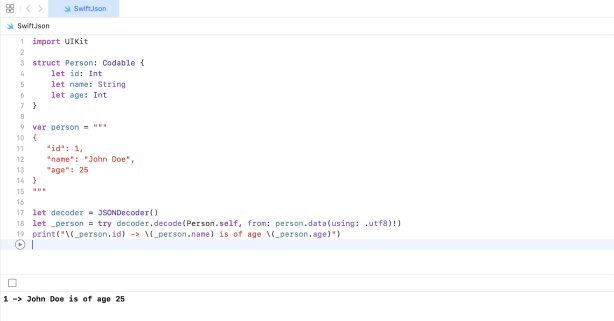
In this code, you’ve deserialized the data to an object and then read the properties as a regular Swift object. The decoder.decode
method accepts the type to which you can translate the JSON document. In this case, that was Person.self
. The same procedure applies to a collection of objects as well. The next section explores how you can use the Contentful Content Delivery API to read the data from your schema for the collections.
You can learn more about the JSON structure on the JSON format website.
Deserializing JSON collections
JSON collections work the same way as objects. To deserialize a collection, you need to use the array type in the Swift type instead of the scalar value. For your person
type, you can change the age value to a roles
field with a type of string array.
The roles field is an array, and the value for this should be a collection in the JSON document using this payload:
The output should look like this:
The scalar and collection values are used interchangeably in a JSON document. A collection can contain an object, and an object can contain a collection of other objects in it.
Content modeling in Contentful
To access Contentful, create a free account. Then, create a content model. Click on the Content model button in the top navigation, and select the Add content type button. This button enables you to create a new content type in the Contentful content platform.

You can create the fields each record for this content should contain on the page. Give a name for this content type and add the fields by clicking on the Add field button in the right bar.
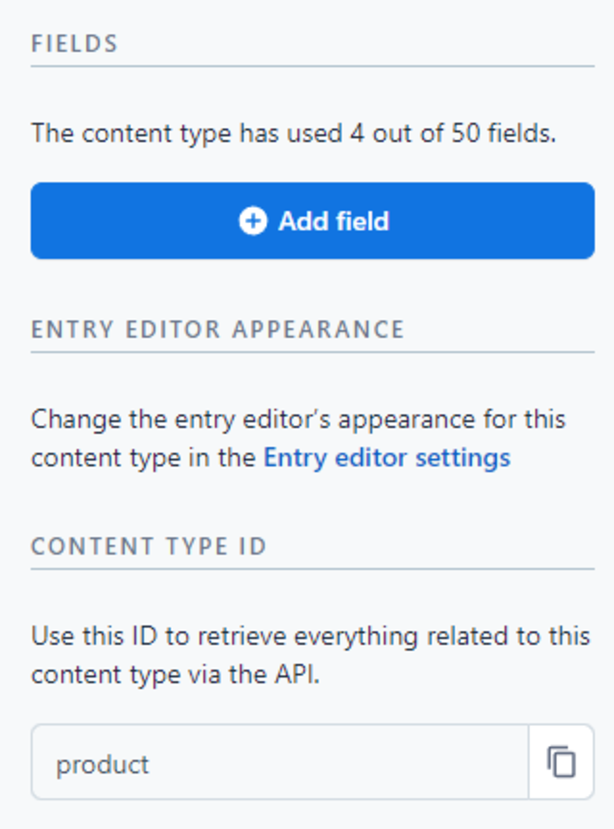
Now save the content model, and create an entry for this content type. You can create content in the product catalog using the Content tab. Click on the Content tab in the top navigation to review existing content and add more content. You can follow the documentation to create another schema or create a record in the database online. Once you’ve created the entries in the Contentful UI, proceed ahead.
The entry that you’ll use later in the article has this structure:
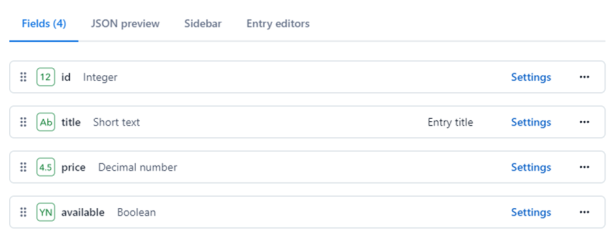
From the top navigation, visit the Content page in your account. You’ll see that you have an entry in the system:
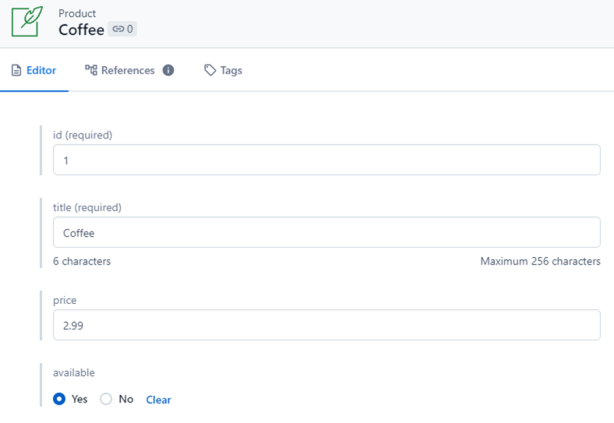
In the next step, you’ll use the Contentful Content Delivery API to query the data using raw HTTP endpoints. You can learn more about the Contentful APIs in our documentation. Find your endpoint using your space ID and provide the access_token
for your account:
tYou can find these values in your Contentful account settings under API keys. You can find the entry-id
, in the Info panel inside of an entry.
You can query this endpoint from your web browser, a cURL interface, or any other HTTP client.
You can deserialize the entire JSON document to an object, or just pick the information that you need to read. For the sake of simplicity, you can deserialize the Fields
data from the document. This field contains the information that your entity has:
Then, you can use the Product type to read the data from the JSON payload. Pass the URL endpoint for your Contentful entries as a string in the URL constructor (replace the _url
below):
The output contains the product instance you created in Contentful:
The content must be updated on the Contentful platform to be reflected on the web applications.
The Contentful platform has SDKs for all major frameworks and platforms, such as JavaScript, Android, .NET, and iOS. It’s recommended to use the SDKs instead of working with JSON yourself. The SDKs provide a better development experience and can enable your teams to get started with the platform quickly. Working with raw JSON documents can help you build custom workflows that might not yet be available in the SDK or specific to your business requirements.
Benefits
The benefits of using Contentful APIs include:
You can build any UI in front of your business’s data.
Your data format is JSON and thus can be used on any platform with any framework.
The JSON payload is lightweight, so it does not consume a lot of network bandwidth.
The JSON payload can represent the version of the data. If your business updates the data more often, you can check the value of the version to perform actions.
Next steps
This article guided you through the basics of JSON format for data interchange and how you can use Web APIs to build dynamic web applications. But this is only one of the many ways you can create with Contentful. Check out our quick start tutorials, developer showcase, or use your free account today to build more.