Choosing the best React CMS
Updated: July 27, 2023
React enables thousands of front-end developers to build fast applications in an intuitive way. Its flexible component mindset changed the back-end developer experience and the way developers build for the web.
What is React?
React is an open-source JavaScript library for building user interfaces (UI) and/or single-page applications (SPA). It was created by Facebook and is used by developers around the world. React allows developers to build complex UIs using a declarative syntax that makes the state of an application explicit.
React uses a component-based architecture, which means that developers break down complex UIs into smaller, reusable pieces of code called components. Each component has its own state, which can be updated using props (short for properties) passed down from its parent component.
React uses a virtual DOM (Document Object Model) to efficiently update the UI when the state of the application changes. Instead of updating the actual DOM, React updates a virtual representation of the DOM and then calculates the minimal number of changes needed to update the actual DOM. This makes React fast and efficient.
React also has a large ecosystem of third-party libraries and tools that can be used to enhance its functionality. It is commonly used in conjunction with other tools and libraries such as Redux and React Router to build robust and scalable web applications.
What are the benefits of using a headless CMS built with React?
A headless CMS (Content Management System) is a content management system that allows you to manage and distribute content across multiple channels, such as websites, mobile apps, and social media platforms, without being tied to a specific presentation layer. When combined with React, a headless CMS can offer several benefits, including:
Customizability | React is a flexible and customizable framework that allows developers to build custom components and templates for content presentation. With a headless CMS and React, you can create a custom user interface that meets your specific requirements. |
Scalability | React is known for its scalability and performance, making it ideal for building large-scale applications. This means that a headless CMS, together with React, can handle large amounts of content and traffic, making it suitable for businesses that need to scale their applications quickly. |
Easy integration | React can be easily integrated with other tools and libraries, such as Redux for state management, React Router for routing, and GraphQL for data fetching. |
SEO-friendly | React's server-side rendering capabilities make it SEO-friendly, as search engine crawlers can easily index the pre-rendered HTML. |
Developer-friendly | React's component-based architecture, declarative syntax, and virtual DOM make it easy for developers to work with the CMS. This can help to reduce development time and costs, as well as improve the overall quality of the codebase. |
Community support | React has a large and active community of developers, with many resources, tutorials, and libraries available to use. This can make it easier to get started with the CMS and to find solutions to common problems, as well as stay up to date with the latest best practices and trends in the industry. |
Overall, using a headless CMS with React can provide businesses with a powerful and flexible solution for creating and managing content-driven applications that meet the needs of their customers. With its scalability, customizability, and SEO-friendly features, a React headless CMS can help businesses stay ahead of the curve and deliver exceptional user experiences.
Why Contentful and React?
Contentful is a cloud and API-first composable content platform that enables you to:
- Define and set up your content structures in minutes.
- Provide your content editors with an interface to create content.
- Query structured data in your React applications via a set of JSON APIs.
All this allows you to make your content reusable — structured JSON works everywhere!
React enables thousands of frontend developers to build fast applications in a more intuitive way. But how do you handle content requirements in cross-functional teams while building apps in the fast-moving React ecosystem?
The Contentful Composable Content Platform enables you to use your favorite tools without getting in your way. Get content creators out of your code base, get rid of maintaining your home-grown CMS servers, and go API-first. Set up your application’s content structures and stop worrying about editors and data that mixes HTML, JSON, and random YAML files.
How a React CMS works
A headless CMS with a React-based frontend — which we’ll refer to as a React CMS — works by separating the content management and presentation layers of a web application.
In a typical CMS, the content management system and the presentation layer are tightly coupled, meaning that the content management system also controls the presentation of the content on the website. This can make it difficult to make changes to the presentation layer without affecting the content management system and vice versa.
In contrast, a React CMS decouples the content management system and presentation layer by providing a headless CMS that can be accessed through an API, allowing developers to build a custom frontend using the React library.
Here's a breakdown of how a React CMS works:
- Content is created and managed in the headless CMS, a capability provided by Contentful, which provides a user interface for content editors to create, edit, and publish content.
- The content is stored in the headless CMS and exposed through an API, such as GraphQL or REST, which allows the content to be retrieved by the frontend application.
- The frontend application, which is built using React components, retrieves the content from the API and renders it on the page.
- Changes made to the content in the headless CMS are immediately available to the frontend application, without the need to make any changes to the application code.
- The React frontend application can be customized and extended using other tools, such as Redux for state management, React Router for routing, and styled components for styling.
Overall, a React CMS allows developers to build custom frontend applications that are decoupled from the content management system, making it easier to make changes to the presentation layer without affecting the content management system.
React components
React’s component architecture is essential for fast, maintainable and scalable frontend applications. Contentful allows you to define content structures that match your components’ requirements. It doesn’t matter if it’s a route component to power pages or a single CTA component. You can quickly connect your code base with your content and start coding!
You’re in charge of defining the content structure for blog posts, authors, or pages.
For example, a blog post can connect to an author and an author can connect to an event. You’re in charge of defining small pieces or big chunks of editable data. You can then fetch a whole content tree in a single API call or receive only specific entries. Request only what you need, shape the API responses to your needs and start interacting with a content graph that goes beyond posts and pages.
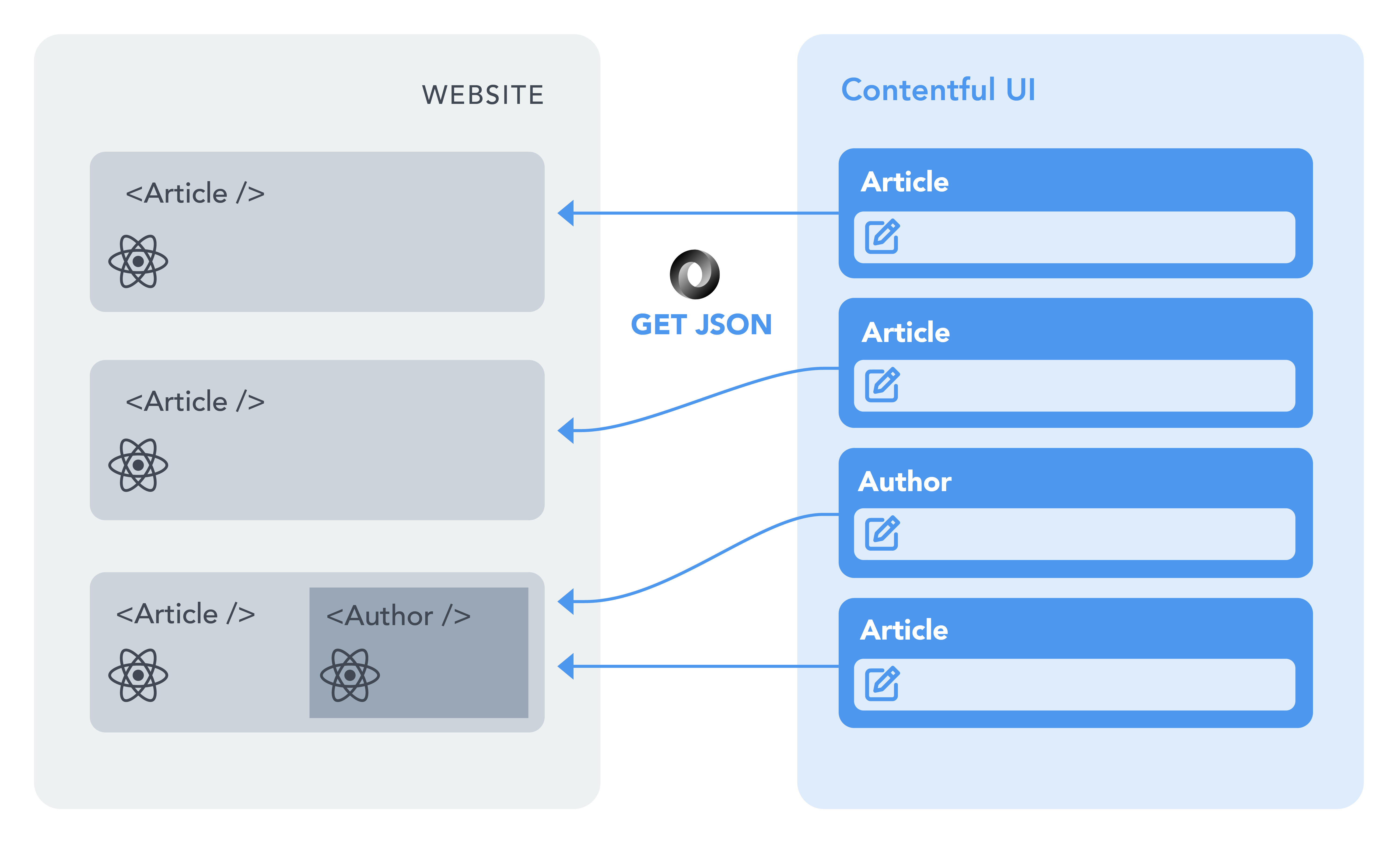
React applications
After setting up your content structures, edit your content in the Contentful interface and fetch it via a set of powerful and fast APIs.
Use the Content Delivery API to request your content via fast CDNs that are ready to handle thousands of requests when your project needs to scale. You can build and enable preview workflows using the Content Preview API, which allows you to fetch draft content and review your application’s content before hitting publish.
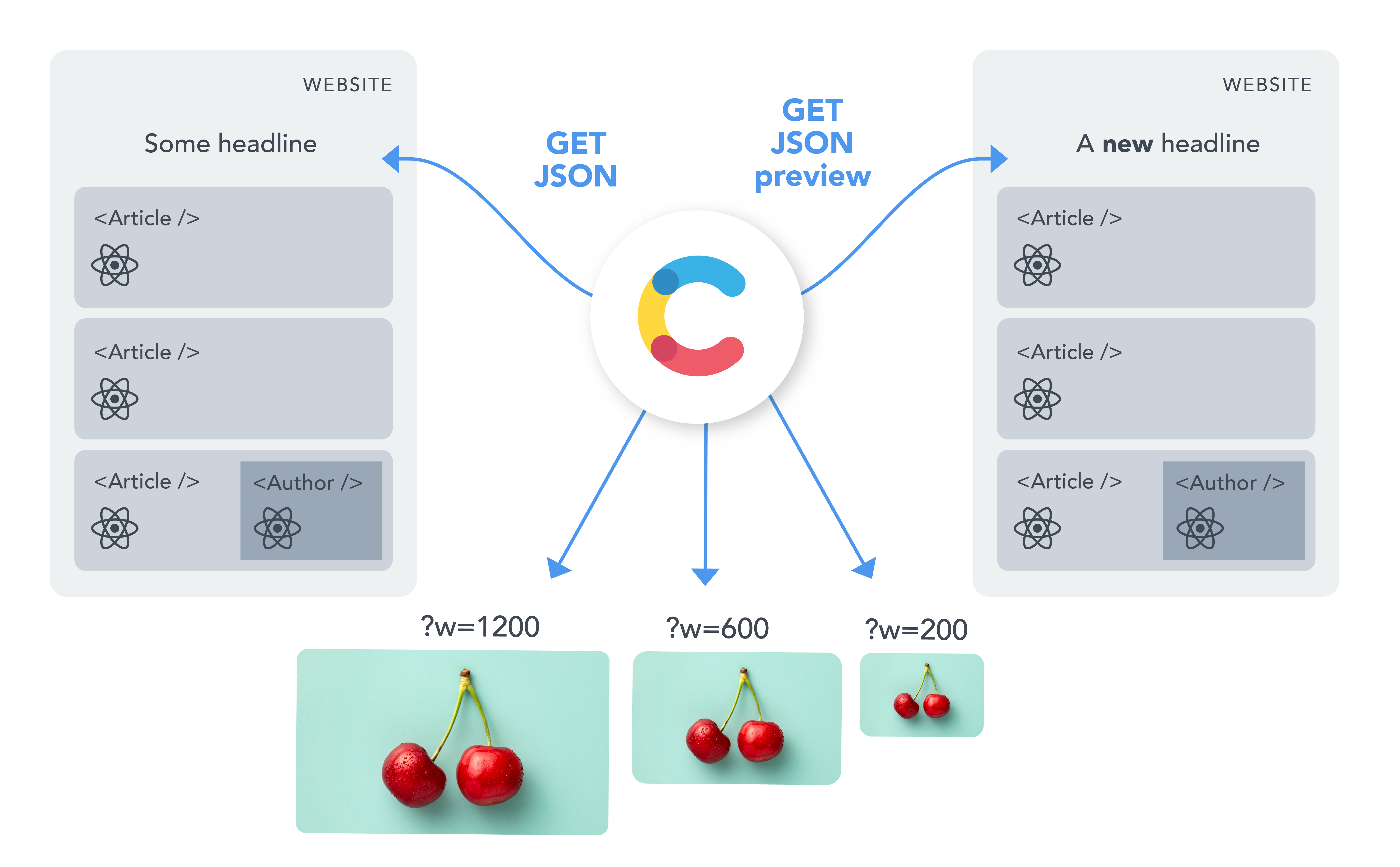
GraphQL and React
And if you prefer, use the GraphQL API to fetch data faster, get predictable API responses, and connect your React components with your content. Make your applications faster: You can query what you need with Contentful as your React content management system.
fetch(`https://graphql.contentful.com/content/v1/spaces/[YOUR_SPACE_ID]`, {
method: "POST",
headers: {
Authorization: `Bearer [YOUR_TOKEN]`,
"Content-Type": "application/json",
},
body: JSON.stringify({
query: `query {
postCollection {
items {
title
}
}
}`,
}),
})
.then(response => response.json())
.then(json => {
const { data } = json;
console.log(data.postCollection.items);
// [ { title: "Hello world"} ]
});
Integrate Contentful into your React application
Since we can’t know your specific editorial requirements, the App Framework exists to make it easier to run your own code and change and customize the editing experience. This guarantees an experience that works for your editors.
If you need custom content validations for a specific entry, or want to enrich the interface with third-party APIs such as your headless ecommerce provider, or build a custom dashboard, use the App Framework to run your React code in the web interface itself. Make the Contentful Composable Content Platform your own and tailor the interface to your needs in your preferred way!
React documentation
Ready to dig into the platform? Sign up for a free Contentful account. Then consult this tutorial to get started with React, which shows you how to connect a create-react-app application with Contentful's GraphQL API. You’ll learn how to use the interface to create a new content type, how to create an entry of this type, and then fetch it from within your new React application using GraphQL. Make with the clicky!
Join our community
Contentful's community includes hundreds of developers and builders sharing what they're working on. To find out more about our community, visit the Contentful Developer Portal, check out our recent developer blog posts, or join our public Slack organization and connect with fellow React developers.