TypeScript vs. JavaScript: Explaining the differences
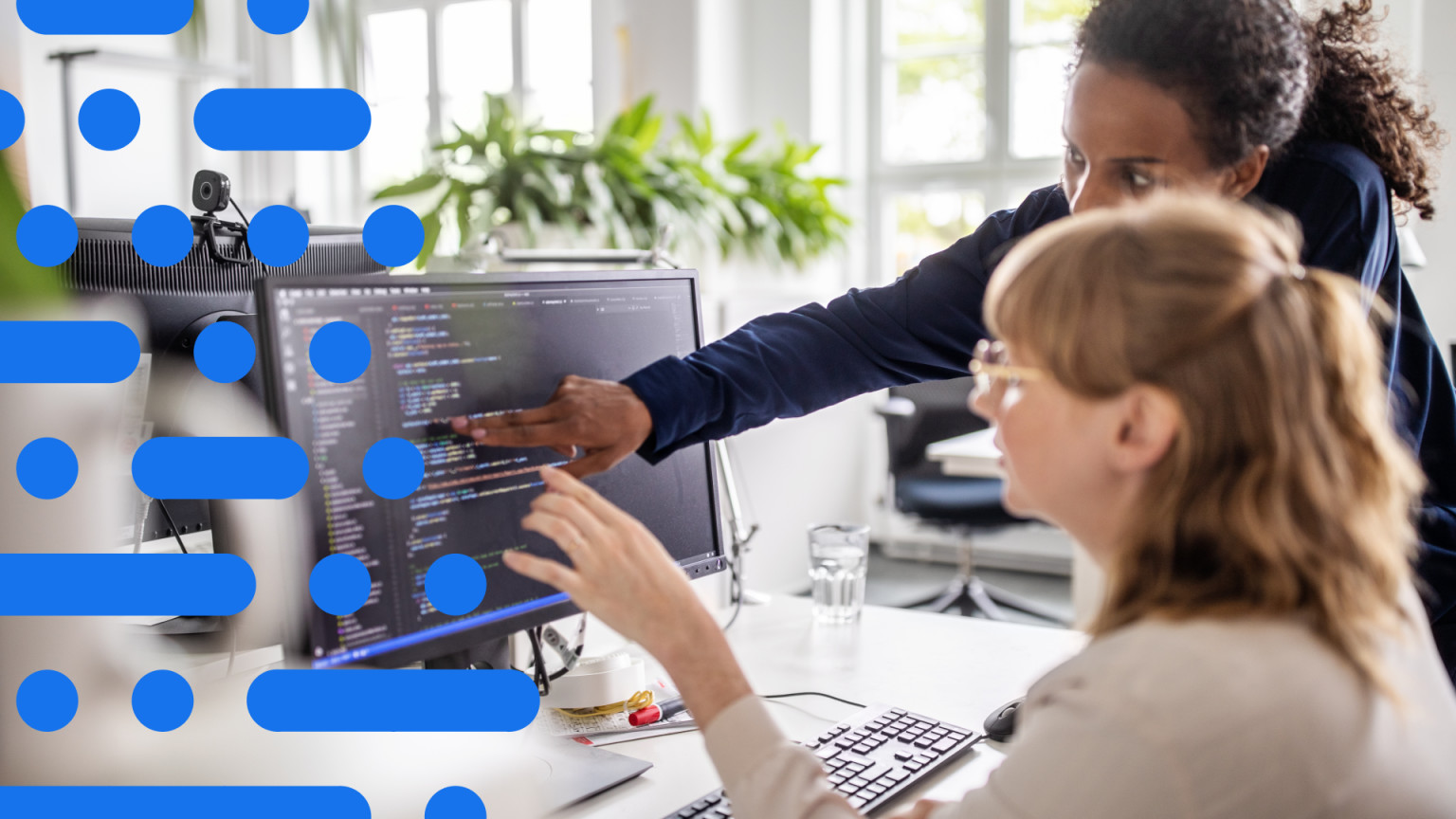
Today, websites are no longer simply landing pages for customers to find a phone number, reference a product, or glance at company information. Websites have been succeeded by web apps which are highly interactive, have intricate and crucial functionality, connect customers to businesses, clients to projects, and products to people.
For most web developers, working on a website means more than working on a simple landing page and it also means working with a team of people who usually work with other teams of people.
Many frontend developers are accomplishing their goals because they can now better identify data types, convey their developer intention, and with the right tools, experience a streamlined developer environment. Traditional frontend JavaScript developers are doing this by using TypeScript.
What is TypeScript?
TypeScript, a project of Microsoft, is a superset of JavaScript that adds static typing to the language. A superset refers to a language that includes all the features and syntax of another language and adds additional features on top of it. In other words, a superset language extends the capabilities of the language it’s based on.
This means that TypeScript includes all the features of JavaScript and introduces additional features like static typing, interfaces, and other constructs not present in standard JavaScript. When you write TypeScript code, you’re essentially writing in a language that builds upon and extends the capabilities of JavaScript with static typing.
What is JavaScript?
Before we explore the differences, the advantages, and the considerations of using TypeScript over JavaScript, let’s back up a little and understand JavaScript itself.
Web developers usually work with three main tools: HTML, CSS, and JavaScript. JavaScript (not to be confused with Java) is a high-level scripting language, widely used and originally known for its role in web development. JavaScript code is primarily used for adding interactivity and functionality to web pages (HTML). It can manipulate the Document Object Model (DOM), making it possible to create dynamic and responsive user interfaces client-side.
JavaScript is either executed on the client’s web browser or on a server-side backend environment like Node.js. Both have dynamic typing which means variable types are determined at runtime (unlike TypeScript which are determined at compile time).
There is a vast ecosystem of JavaScript libraries and frameworks, many of which have TypeScript counterparts enabling more powerful tooling. Let’s look at dynamic typing and compare it to static typing.
Benefits of static typing
For those of us who are coming from a JavaScript world, we may not have seen types before if we aren’t familiar with other languages. However, static typing is a feature that can be found in other languages and has helped developers ship robust code for decades.
What is static typing?
Static typing is a programming language feature that involves explicitly specifying the data types of variables, function parameters, and return values at compile time, before the code is executed. This is in contrast to dynamic typing, where data types are determined and checked at runtime while the program is running.
In a statically typed language like TypeScript, the compiler performs type checking before the code is executed. It ensures that variables are used in ways consistent with their defined types, preventing certain types of errors that can occur due to incorrect type usage.
If a variable’s type is incompatible with an operation, the compiler will raise an error during the compilation process, rather than waiting for runtime (when the user is using your web app) to discover such errors.
Static typing can offer several benefits, including:
Early Error Detection: Type-related errors are caught at compile time, helping developers identify and fix issues before the code is executed.
Improved Code Quality: Type annotations can make the code more self-documenting and easier to understand.
Enhanced Tooling: IDEs and code editors can provide better auto-completion, code navigation, and refactoring suggestions based on type information.
Readability and Maintainability: Explicit types can make code more readable and understandable, especially in larger projects.
Example of static typing
Let’s look at an example TS file that showcases how TypeScript’s static typing helps catch type-related errors at compile time, leading to more reliable and error-free code.
In this code example:
The variable
age
is explicitly declared as a genericnumber
type.The function
add
takes two parameters of type `number` and returns a value of type `number`.Type annotations ensure that only valid types are assigned to variables and passed as function arguments.
If you try to assign a `string` to `age` or call the `add` function with a `string` argument, TypeScript’s static type checking will raise errors during compilation or compile time.
Compile time is the phase where the source code is transformed into executable code and is checked for syntactic and type-related errors, while runtime is when the compiled code is executed and the program's functionality is realized.
In other words, something like: `npm run build` in your npm package is compile time (where the TypeScript compiler/transpiler errors would show up) and a user browsing your site is called runtime (where runtime errors would show up in the browser’s JavaScript console).
Why choose TypeScript over JavaScript?
Developers often choose the advantages of TypeScript over JavaScript because TypeScript offers static type checking, which helps catch errors at compile time rather than runtime like we saw in the example above. This can lead to more robust and reliable code while also minimizing the surface area for code errors. TypeScript supports the latest ECMAScript features while providing enhanced tooling, code navigation, and documentation. It’s especially beneficial for larger projects and teams, as it aids in maintaining code quality and readability.
Factors to consider
The choice between TypeScript and JavaScript depends on factors like project size, team expertise, development speed, etc. Smaller projects with a quick development cycle might opt for JavaScript, while larger, more complex projects could often benefit from TypeScript’s static type checking and tooling advantages due to the following reasons:
Code Maintainability: With TypeScript's static typing, it’s easier to understand the data types of variables, function parameters, and return values. This leads to more self-documenting code, which is crucial for maintaining and extending projects over time.
Reduced Errors: Static typing helps catch type-related errors at compile time, preventing many common mistakes from reaching runtime. This reduces the chances of subtle bugs that can be difficult to track down in large codebases.
Enhanced Collaboration: Type annotations provide clear interfaces for functions and modules, making it easier for team members to understand how different parts of the codebase interact. This leads to smoother collaboration, as developers can work on different parts of the project without stepping on each other’s toes.
Refactoring: Large projects often require refactoring to improve code quality and maintainability. TypeScript’s type system makes refactoring safer by alerting developers to potential issues when they change code. This minimizes the risk of breaking existing functionality.
Tooling and IDE Support: TypeScript developers have access to robust tooling, including code completion, navigation, and refactoring suggestions. These features are invaluable when dealing with large and complex codebases, as they help developers understand the code, debug easily, and make changes more efficiently.
Reduced Documentation Overhead: With clear type annotations, much of the documentation about types and interfaces is embedded directly in the code. This can reduce the need for extensive external documentation and make the code more self-explanatory.
Long-term Maintenance: Large projects often have long life cycles. TypeScript’s static typing and strong tooling support make it easier to maintain, update, and extend the codebase over time, even as team members change.
Code Quality and Consistency: TypeScript encourages a higher level of code quality and consistency across the project by enforcing type standards and providing clearer guidelines for code structure.
Predictable Behavior: In a large team, different developers might be working on different parts of the codebase. TypeScript helps ensure that everyone follows the same coding standards and produces code that behaves predictably.
Better Onboarding: New developers joining a large team can benefit from TypeScript’s type annotations and clear interfaces, making it easier for them to understand the codebase and start contributing more quickly.
With all these benefits it may seem like TypeScript is a no-brainer. However, the choice between TypeScript and JavaScript ultimately depends on the project’s requirements, the team’s expertise, and development priorities.
Smaller projects, rapid prototyping, or teams more comfortable with JavaScript’s flexibility might lean toward using JavaScript. TypeScript is especially advantageous for larger, long-term projects and teams that value strong type safety, maintainability, and collaboration.
Wrapping up
At Contentful, we use TypeScript for our frontend software development to take advantage of all the safety and utility features described above when working on React or Angular projects.
While migrating from JavaScript to TypeScript requires effort and has a learning curve, it’s often a worthwhile investment for larger projects or teams. The transition starts gradually, and the benefits of static typing, improved tooling, and code maintainability become more evident as our software evolves.
If you are looking to integrate with Contentful via our CPA, CDA, CMA, or our App Framework, you will find that using TypeScript with your content model will vastly improve your ability to reason about your own projects and maintain a high level of sanity while developing and shipping code.
For more information, check out the Contentful Developer Documentation or dive into our sections on JavaScript and TypeScript.