How to start automated browser testing with Playwright
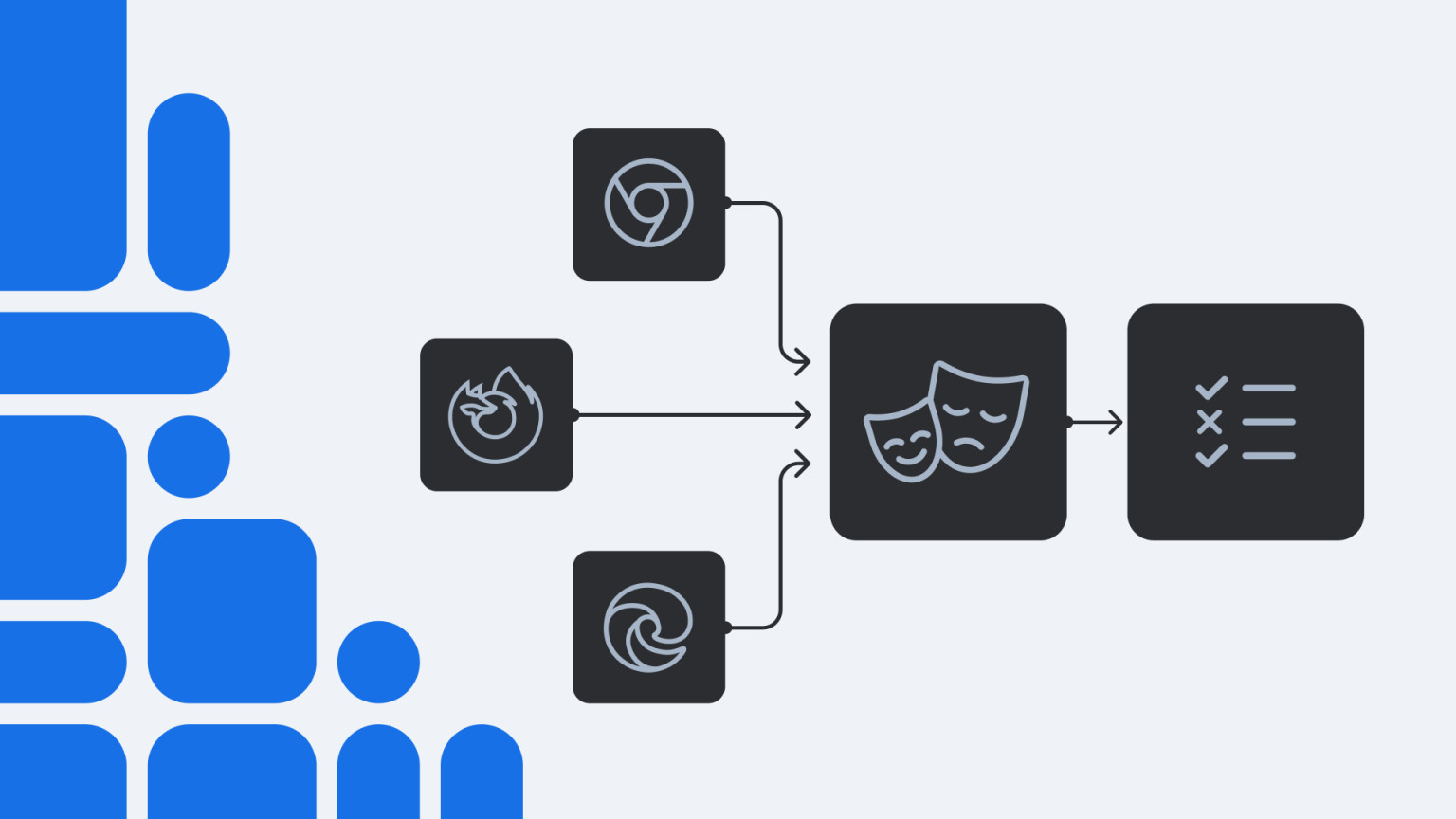
Ensuring an efficient development process and delivering a high-quality user experience require developers to rigorously test their frontend applications after each update. This post explains what Playwright and end-to-end browser testing are, how browser testing aids your development process, and shows you how to implement Playwright automated testing in your projects.
What is Playwright?
Playwright (sometimes referred to as Playwright.js) is an end-to-end web testing tool that abstracts away the complexity of testing web frontends on different browsers and screen sizes. It has thorough documentation, strong community support, and a focus on the developer experience, with features such as expressive test syntax and native packages for TypeScript, JavaScript, Python, .NET, and Java.
Web testing, also known as end-to-end testing and browser testing, is the automated process of making sure that a web page or application appears and functions as intended by rendering the application and simulating user interactions like clicks and keypresses. As scripted interactions run the results, the results are checked against a set of rules, and the developer is notified of any failures — removing the need for manual testing of different environments.
Playwright runs its tests in a headless browser, so it works with any frontend framework, including Angular, React, Vue.js, or just plain old HTML and JavaScript.
Offloading tasks like browser testing lets you focus on building great applications. In combination with composable content, automated end-to-end testing with Playwright can greatly reduce the amount of work required to deploy and maintain large-scale, media-rich, and cross-channel applications.
Automated web testing with Playwright vs. Selenium, Cypress, and Puppeteer
In addition to Playwright, there are several established browser testing tools such as Selenium, Cypress, and Puppeteer, each with its own benefits to developers with different requirements. Playwright has become increasingly popular due to its modern features and ease of use, building on the developer experience provided by earlier end-to-end testing tools.
Ease of use
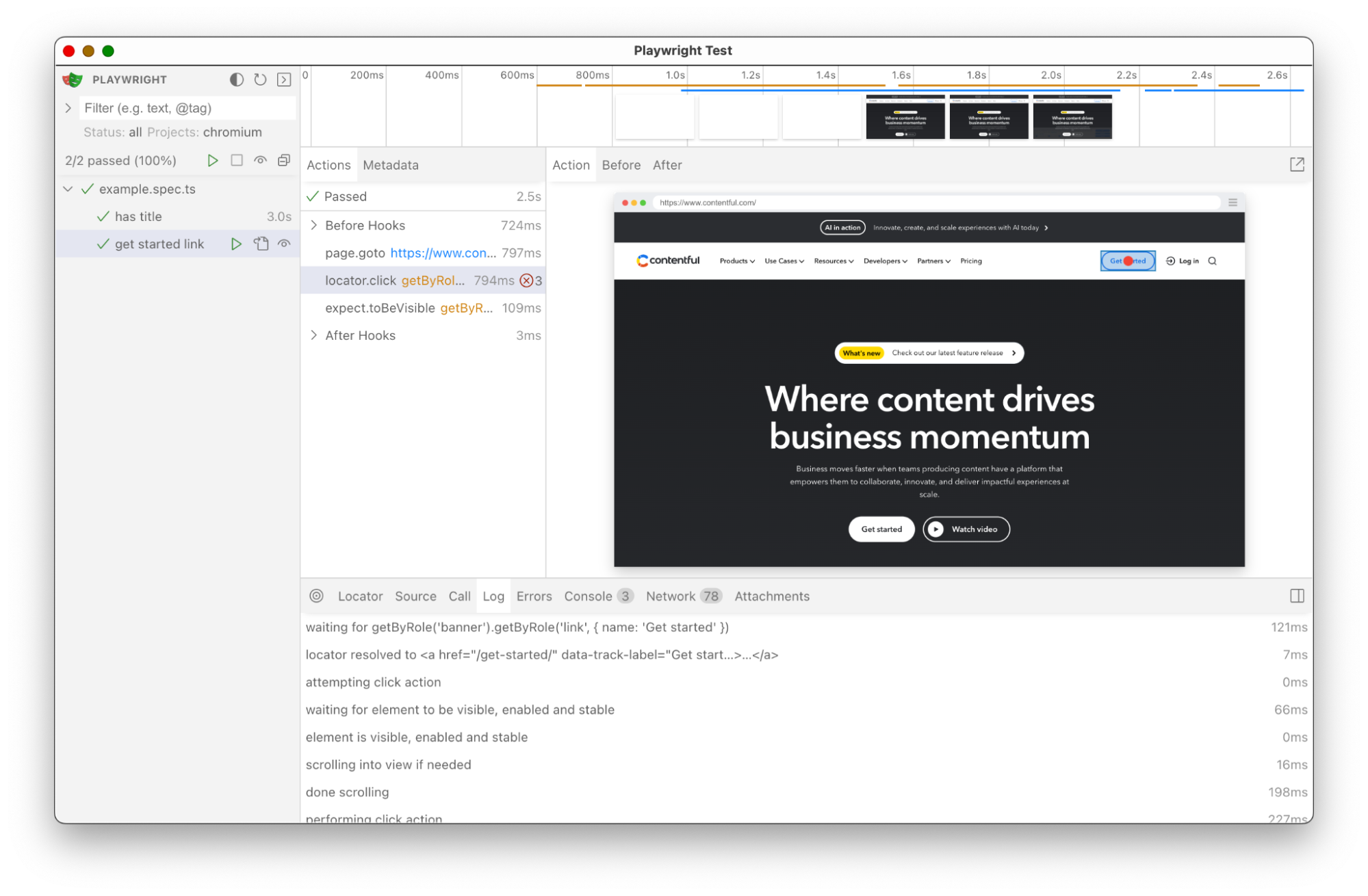
Playwright is designed to integrate with your existing development tools and workflows, with support for several languages and a Visual Studio Code plugin. It has an understandable syntax that makes the purpose of each interaction clear, and a UI mode that allows you to run, watch, and debug tests.
Playwright | Selenium | Cypress | Puppeteer | |
---|---|---|---|---|
Visual Studio Code plugin | No | |||
Graphical user interface | ||||
Supported languages | TypeScript | TypeScript |
Playwright, Selenium, Puppeteer, and Cypress are all open-source projects that are free to use. Cypress locks some functionality behind a paid enterprise subscription, while the other solutions are all 100% free with active online communities.
Unique to Playwright are their extensive release notes that include video explainers. This is super helpful for developers who want to get up to speed with the practical implications an update will have on their workflows.
Speed
Being able to run tests quickly after each code update results in faster development and less waiting. This requires both fast execution and being able to run tests in different browser environments in parallel.
Playwright has a documented speed advantage over Cypress, Selenium, and Puppeteer and allows you to run tests using multiple browsers at the same time.
DevOps and testing features
Playwright requires little initial configuration, providing a ready-to-use testing environment while still offering flexibility in how you construct your tests.
Playwright | Selenium | Cypress | Puppeteer | |
---|---|---|---|---|
Run tests in a Docker container | ||||
CI/CD integrations | Manual | Manual | ||
Selenium Grid integration for running tests on multiple machines | Yes | No | No | |
Supported OS | MacOS, Linux, Windows | MacOS, Linux, Windows | ||
Supported testing browsers |
How Playwright testing works: browser contexts, pages, tests, and traces explained
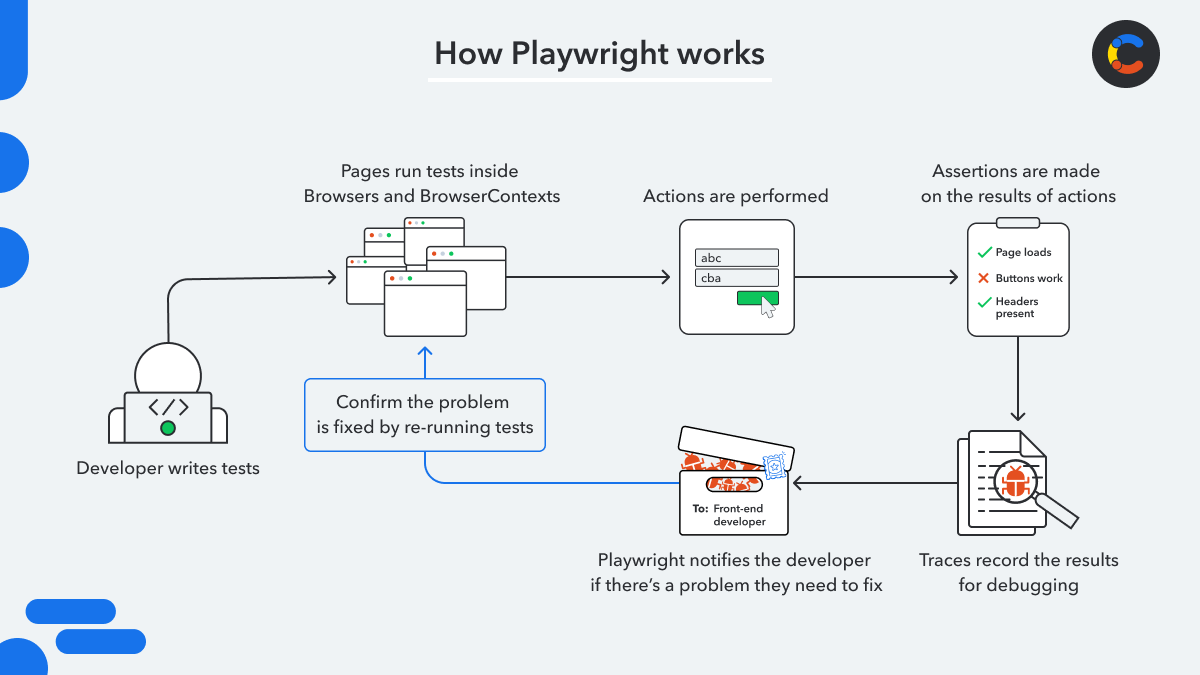
Playwright consists of a few moving parts that you should understand before getting started:
Browsers and BrowserContexts: Browsers are the web browser applications Playwright uses to test applications, including Chrome, Firefox, Edge, and Safari. BrowserContexts allow you to run tests in multiple isolated browser sessions at the same time.
Pages: A page is an individual tab or browser window within a BrowserContext. Each page is used to load documents, traverse links, and interact with content.
Tests: Tests perform actions and make assertions against the result of those actions. If an assertion does not match an expected value, the test will fail.
Traces: Traces record information as your tests run for debugging purposes. If a test fails, you can use the data stored in the trace to find out what went wrong.
Locators: Locators find a page element using a DOM selector. Playwright can then check these elements with assertions or interact with them using actions.
Navigations: Navigations allow Playwright to traverse your website and move from page to page while running tests.
This covers the basic concepts required to start building tests. The Playwright documentation provides a complete reference to all of the functionality, as well as fully documenting the Playwright API, usage in a number of programming environments, and testing best practices.
Guide: Getting started with Playwright.js end-to-end testing
To get started with Playwright for both debugging and automation, you'll first need to install it according to the instructions for your platform.
If you're using npm, navigate an existing project or create a new directory for it and then install Playwright by running:
npm init playwright@latest
You can also get started by installing the Visual Studio code extension.
Testing your Playwright installation
To test that Playwright is installed and available in your project, run the example test command:
npx playwright test
The example test will output a HTML reporter by default. To view the last generated report, run:
npx playwright show-report
You can also run the test and view the results using Playwright’s UI mode:
npx playwright test --ui
Writing Playwright tests
Playwright allows you to write tests in a number of different environments (including Python, Java, and .NET). The default is TypeScript and Node.js.
Tests should be stored in the ./tests
directory in your project and follow the naming scheme your-test-name.spec.ts
. A “spec” or specification encapsulates the expected behavior of a page or set of interactions, and usually consists of multiple tests.
Navigating pages and checking page elements
Below is a Playwright test that checks that a heading exists on a page:
This example test, named ”has hello world heading,” navigates to the page at the specified URL and then uses the getByRole()
locator to find the page element that is to be tested by its role and accessible name (in this case, “Hello World!”). The expect function makes an assertion: if the element exists and the result of the toBeVisible() test is truthy, the test will pass.
Interacting with the page
You can chain multiple assertions and interactions together, letting you build out your tests to simulate what you expect a user to do on the page, including clicking, typing, and interacting with forms. For example, you can check that a user can fill out a comment form:
The above test navigates to a page, fills out a comment box, clicks the submit button, and then checks that a success message appears.
Test isolation via a new browser context
You can create as many tests as you require. Playwright will run each one in a separate, isolated browser context (BrowserContext in Playwright terms):
Generating tests
You can generate tests instead of manually writing them by running the following command:
npx playwright codegen example.com/myblog
You can then perform actions in the web browser yourself and have them recorded as Playwright tests automatically.
Running tests
Use the test command to run Playwright tests:
npx playwright test
You can also run tests in UI mode:
npx playwright test --ui
You can run tests for a specific browser using the --project
flag:
npx playwright test --project firefox
You can run specific tests by specifying the filename:
npx playwright test test-blog-post.spec.ts
Using test results to debug
To debug your code, you’ll need to inspect the results of a failed test, update your code, and re-run the tests. By default, Playwright saves reports using the HTML reporter, and you can configure additional reporters depending on your requirements (for example, some reporters are more human-readable, while others are suited for CI integration).
The most user-friendly way to view test results is using the Playwright Trace Viewer that lets you visually inspect the results of each test.
Using Playwright automated testing to improve product reliability and accessibility
Once you've built your testing workflow in Playwright, you can take your testing automation further by integrating it with your CI/CD pipeline so that tests run automatically whenever new code updates are pushed to your Git repository.
End-to-end testing and CI/CD aren't limited to automated testing for your code updates. Modern, cross-channel digital publishing requires a separation of code and content so that creatives can create and revise content without having to go through the tech team every time new assets need to be uploaded. Composable content platforms enable this, allowing you to use the same content across different channels: for example, publishing a product announcement that appears both in apps and on websites using the same assets.
Automated testing of content updates using end-to-end browser testing tools like Playwright that can be integrated with composable content platforms ensures that nothing breaks on the frontend. Newly published content can be checked for problems like broken links and missing media, and tested to make sure it doesn't interfere with other important page elements like navigation.
You can also test the accessibility of your content using Playwright to ensure that your customer-facing content is available to those who use assistive technologies like screen readers. Keeping your websites, apps, and content WCAG compliant demonstrates a commitment to equality and makes sure that your content reaches the largest possible audience.
Using the right tech stack streamlines the creation of amazing user experiences
The effectiveness of your development process and its outcomes depend on the tools you choose to use. Even talented development teams can see huge gains to their productivity and the quality of their products by choosing tools that let them focus on what matters — their end users.
Integrating Playwright automated browser testing into your development process reduces the amount of time spent testing your frontends, allowing you to focus on keeping your platforms up to date with the latest trends and expectations.
Similarly, building with a composable content platform like Contentful allows developers to focus on realizing and iterating on their ideas, rather than building out backends and manually publishing content updates. You can create apps and websites with all of your own bespoke text, images, and other content, created and curated to your creative teams.