GraphQL vs. REST: Exploring how they work
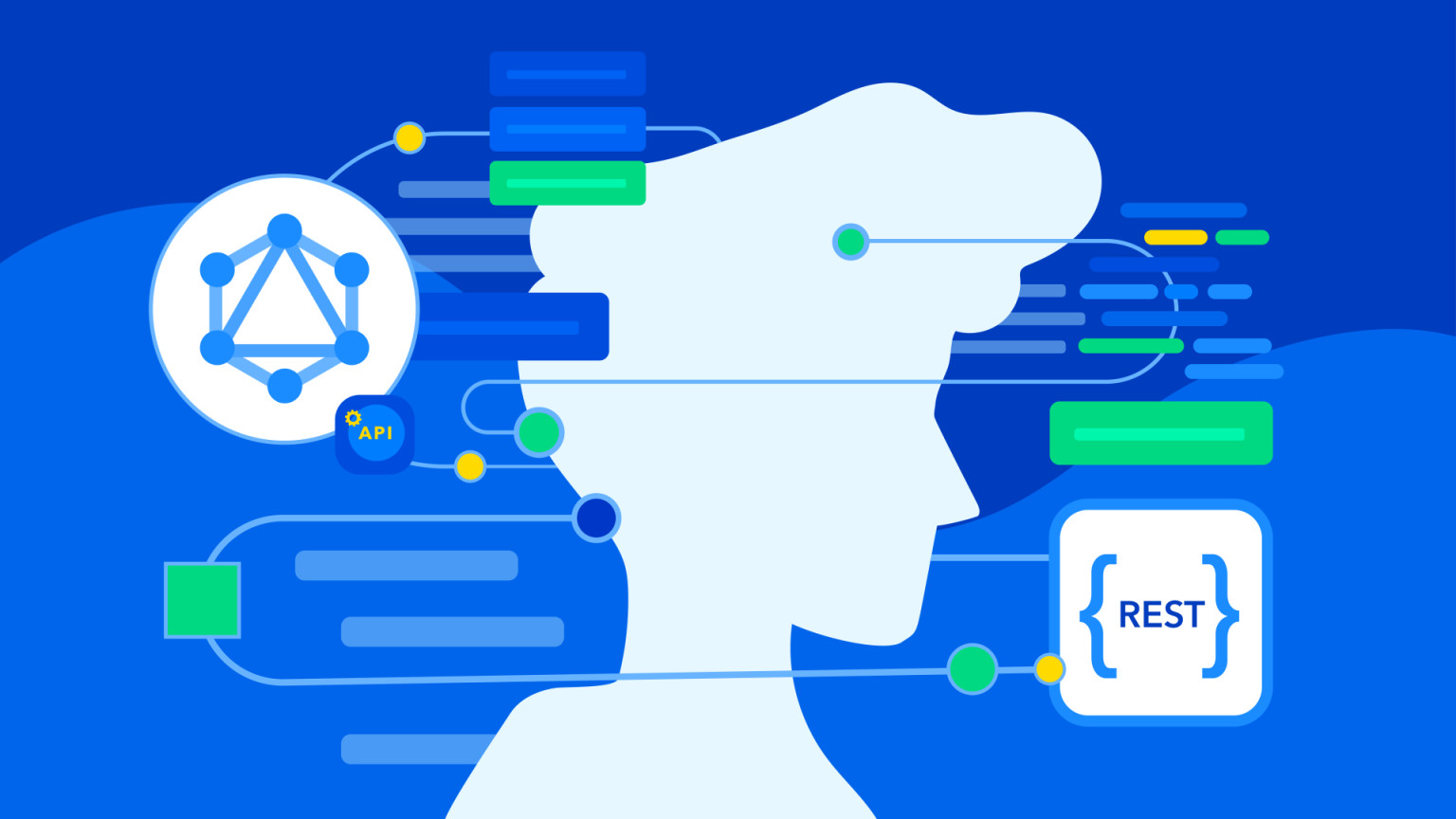
GraphQL and REST are two different approaches for building APIs. The main difference between them is in how they handle data retrieval. In this post, we’ll take a closer look at what they are, with some examples to demonstrate how each of them works.
What is REST?
REST (an acronym for REpresentational State Transfer) has been the standard way of creating APIs for a while. It was created back in 2000 by Roy Fielding (one of the authors of HTTP) for his phd dissertation. REST APIs are defined as "an architectural style for distributed hypermedia systems." It’s surprisingly hard to define what REST is and isn’t, but we’ll keep things high-level.
Here are the main ideas of REST APIs:
No transient state is kept by the server between requests (hence, state transfer).
Endpoints are explicit. More on that later.
HTTP “verbs” like
HEAD
,GET
,POST
,PUT
,DELETE
, andPATCH
explain what’s happening to the data.
As it’s written in the dissertation, "Each request from client to server must contain all the information necessary to understand the request, and cannot take advantage of any stored context on the server." Caching is also mentioned multiple times in the original document.
If you want more details and examples, feel free to read this article.
Let’s see what the most minimal api call with a REST API looks like. With curl
on any unix-based computer, this is all you have to do:
curl "https://api.github.com/users/ruby"
One of the key ideas with REST APIs is that by looking at the endpoint alone, you know what kind of response you’ll receive. In the example above, you’ll receive a response from the GitHub API about a user with the username ruby
.
REST resources
REST has a concept of "resources" which essentially means any entity in the API. In general, we have one endpoint for one resource.
Take GitHub's REST API for example:
A user is a "resource". We can interact with this resource by making a GET request to
https://api.github.com/users/{username}
. We replace{username}
with the actual username of the user we're interested in. There’s no other way to get a user’s info.Similarly, a repository is also a "resource". We can get info about a specific repository with a
GET
request tohttps://api.github.com/repos/{owner}/{repo}
, replacing{owner}
and{repo}
with the respective values.
Benefits of REST
It’s all in the URL: Reading the URL gives you information about what you’re doing.
Standards-based: It relies on standard HTTP methods and status codes.
Caching: Rest on the shoulders of the decades of work on HTTP caching.
Disadvantages of REST
Over-fetching or under-fetching data: Clients might receive more data than they need (over-fetching) or might need to make multiple requests to fetch all required data (under-fetching).
Versioning: When the API evolves, it may require versioning to maintain backward compatibility, (hence the
/v1/
that you see in URLs).
What is GraphQL?
GraphQL is a JSON-like language that was invented in 2012 by Facebook. The company used it internally for three years before making it open-source in 2015. In 2019, the ownership was transferred away from Facebook to the GraphQL Foundation.
GraphQL is a type of API that works via its own query language (that’s where the QL bit comes from). Unlike REST, which can be a bit nebulous, GraphQL is a full-featured language with a specification, just like SQL.
Here’s an API call, with GraphQL, still using curl:
As you see, it’s just HTTP, there’s no need for a library or framework to use GraphQL.
A few things jump out:
With GraphQL, the request is longer but more explicit.
The important information about the request is in the query, rather than in the URL, like in REST.
The URL stays the same, and the endpoint is different. It’s rare to see both GraphQL and REST use a single endpoint. With our Contentful API, the REST API is on https://cdn.contentful.com and the GraphQL API is on https://graphql.contentful.com.
If you unroll the payload of the API call above, you get this:
Graph Query Language
As mentioned above, GraphQL is its own query language. No one wants to learn a language just to make API requests, but this one is designed to get out of your way.
GraphQL is a language designed for both frontend and backend developers. To me, most of the learning curve came down to the jargon (you'll hear about mutations, resolvers, etc). But at the end of the day, if you can write JSON, you can write GraphQL.
Here’s the GraphQL query again, taken out of the previous API call.
GraphQL comes with a sweet tool to help you write it: The GraphQL Playground.
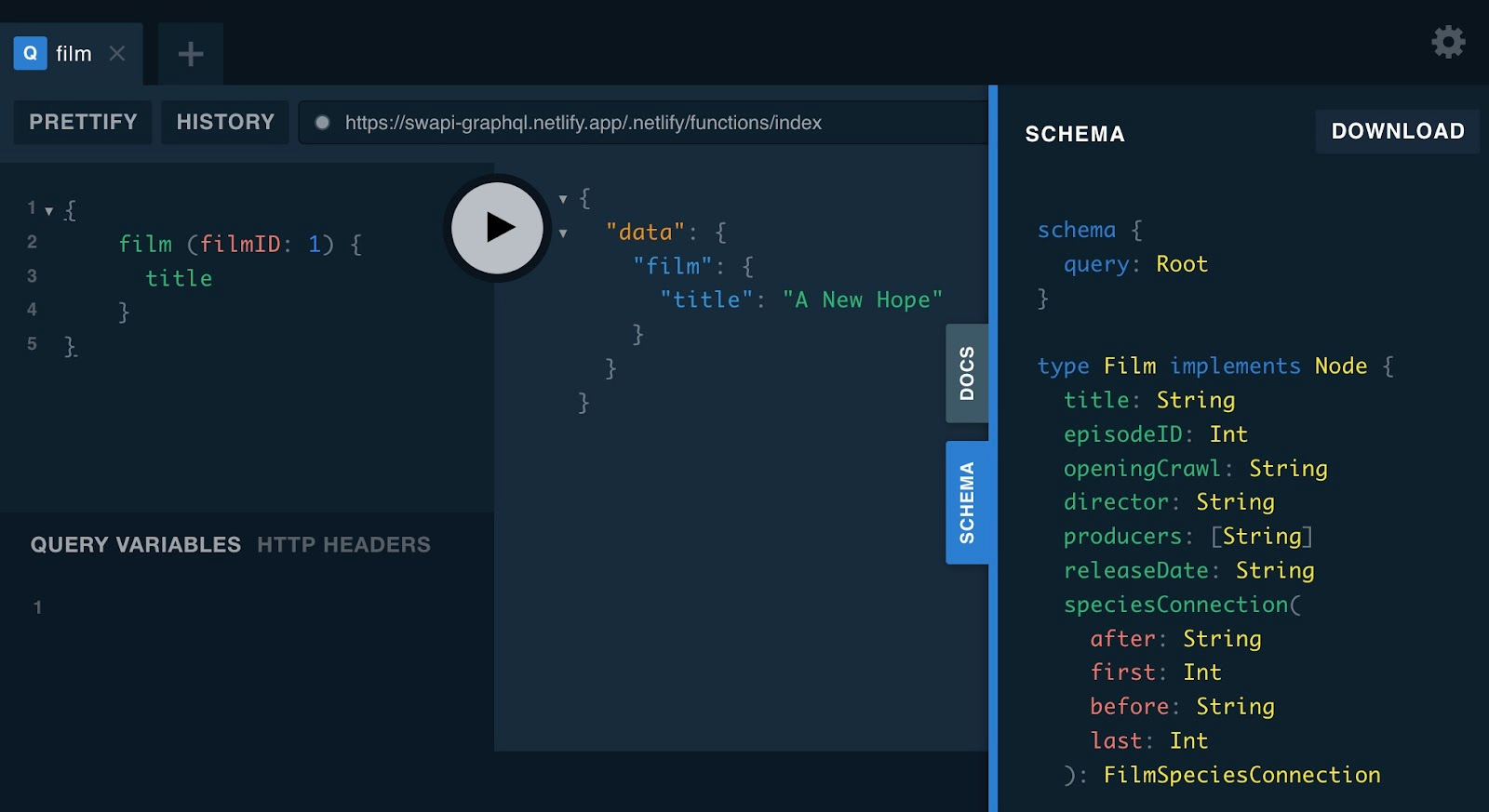
This tool gives you autocompletion, lets you send requests and see responses directly in one window. Similarly what Postman gives you with REST. (To be clear, Postman also supports GraphQL.)
Companies with GraphQL APIs often provide their own playground. Here at Contentful, our playground is at this address. GitHub also has one here, and so on.
The screenshot above is from a nifty website called GraphQLBin, which lets you create a playground from any public GraphQL endpoint.
Benefits of GraphQL
Flexibility: Clients can request exactly the data they need, which reduces over-fetching and under-fetching issues.
Single request: A single GraphQL query is all you need!
Evolution: Changes to the server schema do not necessarily require clients to be updated.
Disadvantages of GraphQL
Complexity: Implementing a GraphQL server can be more complex than a REST API, especially when dealing with complex data models.
Caching: Because requests are more specific, and thus more unique, caching in GraphQL is more challenging to implement.
Comparing REST and GraphQL using, er, droids
Let’s have some fun. In this example, we’ll use the unofficial Star Wars API, which has both a REST and a GraphQL endpoint, to fetch a list of all the droids in the galaxy.
It’s a bit of a contrived example, but it’s on purpose. It's purely to compare how the two technologies fare. In this API, the droids are classified as “people”
and have a species id of 2
.
Finding the droids you’re looking for with REST
In JavaScript with native fetch in Node.js v18 or using node-fetch
, we can grab a list of all the droids in the API like this:
With this approach, you would get something similar to this:
Poor BB-8.
Finding the droids you’re looking for with GraphQL
With GraphQL, you’re not constrained by the concept of “one endpoint, one resource;” you only need to make one request, so here’s the query:
And here is how you'd make that request in Node.js
You do not need an npm package or a framework to make a GraphQL request.
The GraphQL version would return data like this:
So, how did they do?
As you can see, both REST and GraphQL can achieve the same result, but GraphQL does it in a single request, which makes the client-side logic a lot simpler.
I want to mention, again, that you don't need an npm package, a framework, or anything else to work with a GraphQL API. If you can make an HTTP call, that's all you need. It works in most programming languages.
REST = Relational
A good proxy to think about "GraphQL vs. REST" is to think of relational and graph databases.
REST behaves like a relational database, data is organized into tables. Here's a rough diagram:
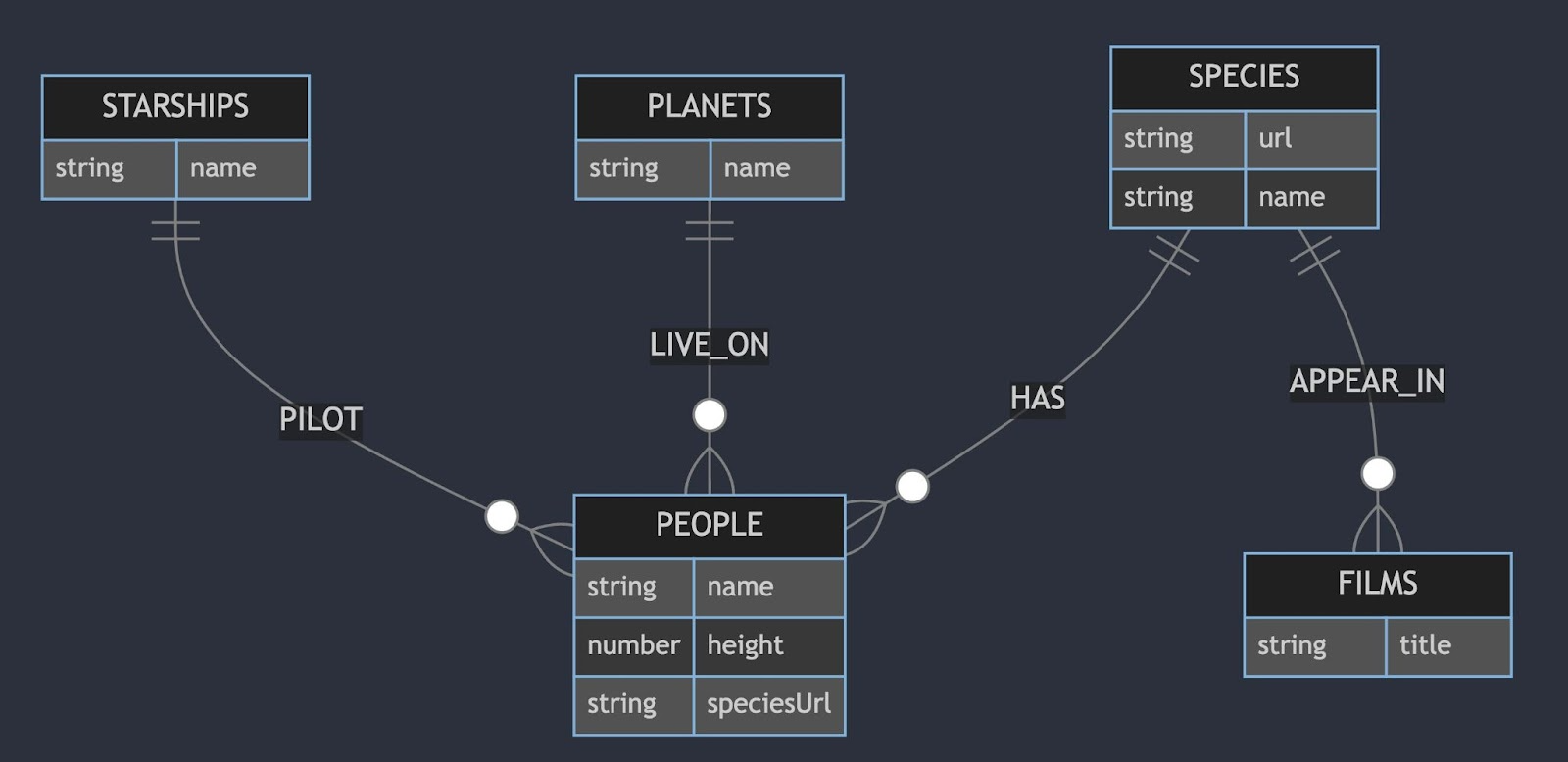
On the other hand, GraphQL is like a graph database, where data is represented as nodes (entities) that are connected by edges (relationships).
Here's another rough diagram:
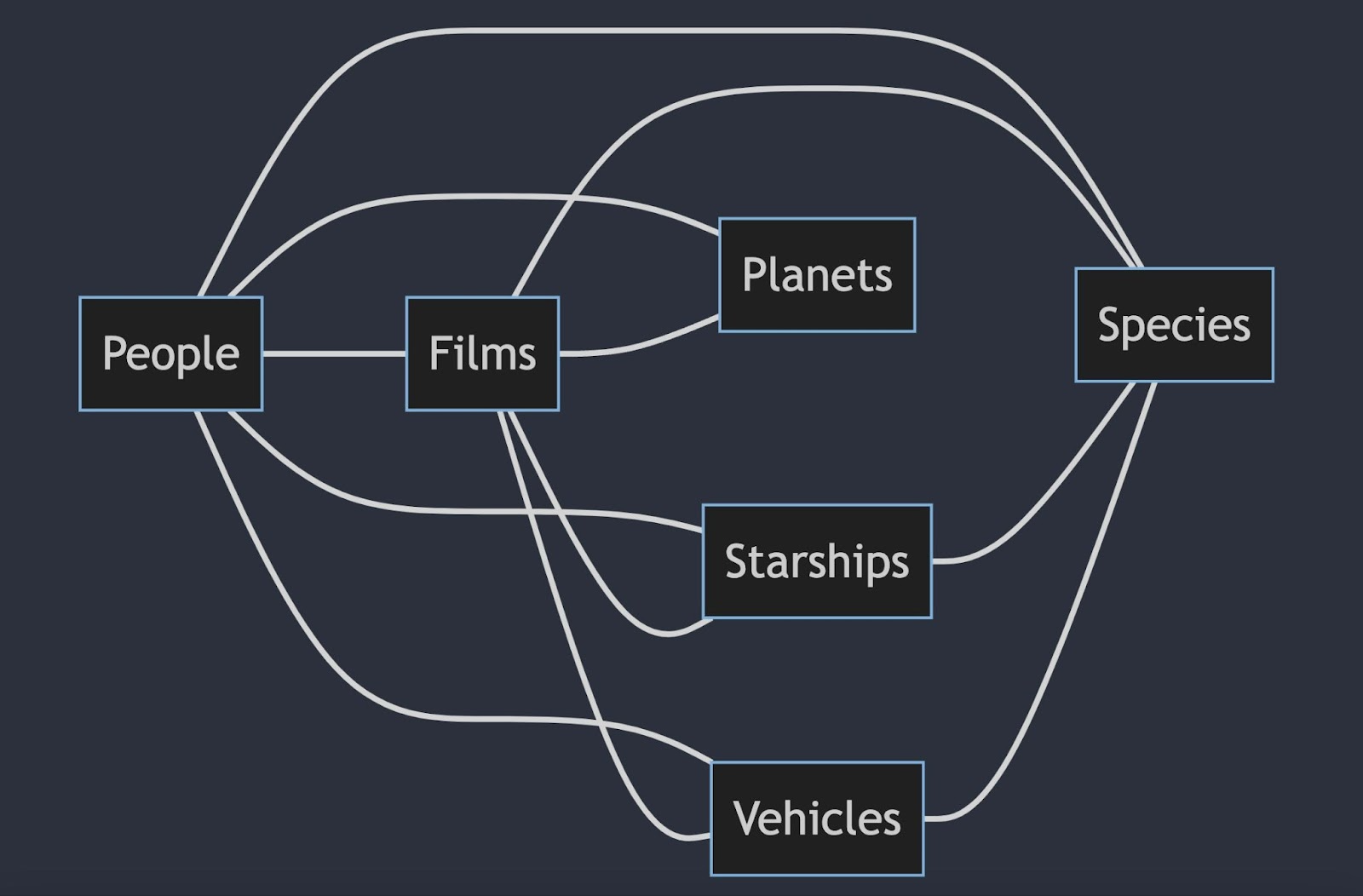
This structure lets you fetch data directly because nodes can be traversed in a single query.
Like in traditional databases, the list of entities and their relationship is called a GraphQL schema.
Wrapping up
In summary, the big deal about GraphQL is how explicit it is. It allows clients to have more control over the data they get and reduces “over-fetching.”
As always with data formats, the choice between GraphQL and REST depends on your specific use cases.
Good news! Contentful gives you both a REST API and a GraphQL API so you don't have to choose.