How to get started with our JavaScript SDK
Introduction
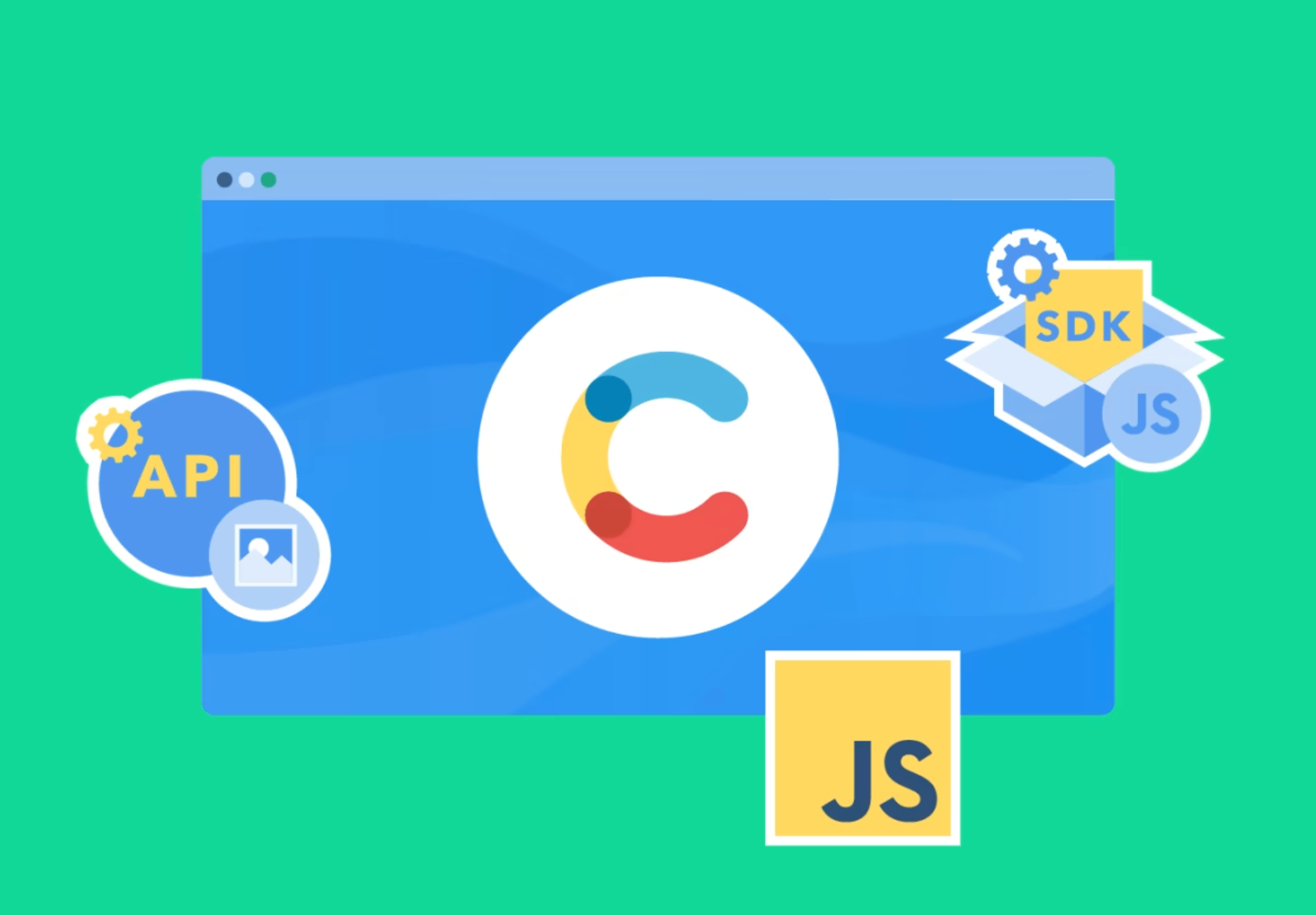
Every time we attend a Meetup or speak at a conference and the topic of content management systems comes up, we hear the same thing time and time again.“I don’t want to deal with that stuff.”The wording may be a bit more graphic than that, but the sentiment is still the same. When JavaScript developers set up a Content Management System (CMS), they’re sick of the burdens that come with managing self-hosted systems that need to be updated every week. This includes worrying about database architecture, scalability issues, and other DevOps protocols. That’s why we’re proposing a new solution: an API-first, technology agnostic content platform.In this article, we’ll highlight the benefits of using Contentful and a content platform for your next JavaScript project.
What is Contentful?
Before we go any further, it’s important to clarify what you’ll be getting with Contentful. Through our content platform, you’ll be able to enable developers to fetch any type of digital content with API calls, while offering editors a familiar-looking web app for creating and managing content. Unlike a CMS, we give you freedom to create your own content model, which allows you to define the data structures you need. From there, we provide you with RESTful APIs so you can deliver content across multiple channels—from websites and mobile apps to IoT, virtual reality games, or anything else you can imagine. A popular use case for a content platform is web apps that use JavaScript frameworks such as React, Angular, and Vue.js. Or websites built with a static site generator.These ties to the JavaScript community are no coincidence. Our content platform is a great match for any JS developer because they allow for more creativity than traditional content platforms. This is because there’s no front end structure attached. It’s simply a backend delivering content through a RESTful API, so you can make it look and feel however you want. And you can choose whatever hip stack you’d like—your data is just one Ajax call away.
Getting started with our JavaScript SDK
We provide SDKs for popular programming languages like JavaScript, Ruby, PHP, and more. These SDKs are designed to make your life as a developer easier when using Contentful and give you almost instant access to our APIs and their features. Another huge advantage to our JavaScript SDKs specifically is that they are universal, meaning that they’re built to work in a Node.js environment and in browsers.Now, let’s see what this looks like. To get the Content Delivery API (CDA) SDK, you have two options. You can install the npm package and import it into your code:
How to get started with our JavaScript SDK / CS1
const contentful = require('contentful');
Or use the pre-built and minified JavaScript file from a CDN:
How to get started with our JavaScript SDK / CS2
<script src="https://unpkg.com/contentful@latest/dist/contentful.min.js"></script>
Then, you can work with the following code snippet to fetch content from Contentful:
How to get started with our JavaScript SDK / CS3
const client = contentful.createClient({
// This is the space ID. A space is like a project folder in Contentful terms
space: 'developer_bookshelf',
/* This is the access token for this space. Normally you get both ID
and the token in the Contentful web app */
accessToken: '0b7f6x59a0'
})
/* This API call will request an entry with the specified ID from
the space defined at the top, using a space-specific access token. */
client.getEntry('5PeGS2SoZGSa4GuiQsigQu')
.then((entry) => console.log(entry))
A treat for front-end developers: Images API
Along with providing true separation between content and presentation, there’s another aspect that draws in front-end developers to Contentful: the Images API.Our Images API allows the retrieval and manipulation of image files references from assets. Once you retrieve an unmodified image, you can then resize and crop it, change its background color, and convert it to different formats just by appending a query parameter. Shipping responsive images in the correct size using our API helps you deliver exactly what you need and save data on the wire in the process.For even more details on what is possible, read the reference documentation for the Images API.
Integrating Contentful with your Node.js backend
When you’re using Contentful, you’re in good company. We’re developers ourselves, and we understand that even if you love all things server-side, you probably don’t want to waste time configuring a JavaScript CMS on your own.Contentful is designed to get you up and running within minutes. You can create and deploy a project that fetches data from a Node.js server-side in just five minutes. We also have a number of Node.js backend tutorials to help you take your first steps.To get you started, we set you up with a demo project based on Contentful’s ‘Product Catalogue’ template so you can see it for yourself. Once you have Node installed, just run the following commands:
How to get started with our JavaScript SDK / CS4
git clone https://github.com/contentful/contentful_express_tutorial.git
cd contentful_express_tutorial
npm install
npm run dev
And there you have it, you should now be able to view this page by opening https://localhost:3000 in your browser. To begin adding and using your own content, you can follow the rest of the steps in this tutorial on creating an Express JavaScript application with Contentful.
What’s next
We encourage you to check out the Contentful for JavaScript page for tutorials, example apps, and more. Or if you’re ready to jump right in, create a free account and start interacting with our content infrastructure today.